How to develop a RESTful webservice in java ?
How to create my first RESTful web service project in Eclipse ?
1) To Create a web service project, open File menu à New à Dynamic Web Project
2)
2) Type the name for your project as “MyFirstRESTful” and select dynamic web module version as 2.5 à Next à
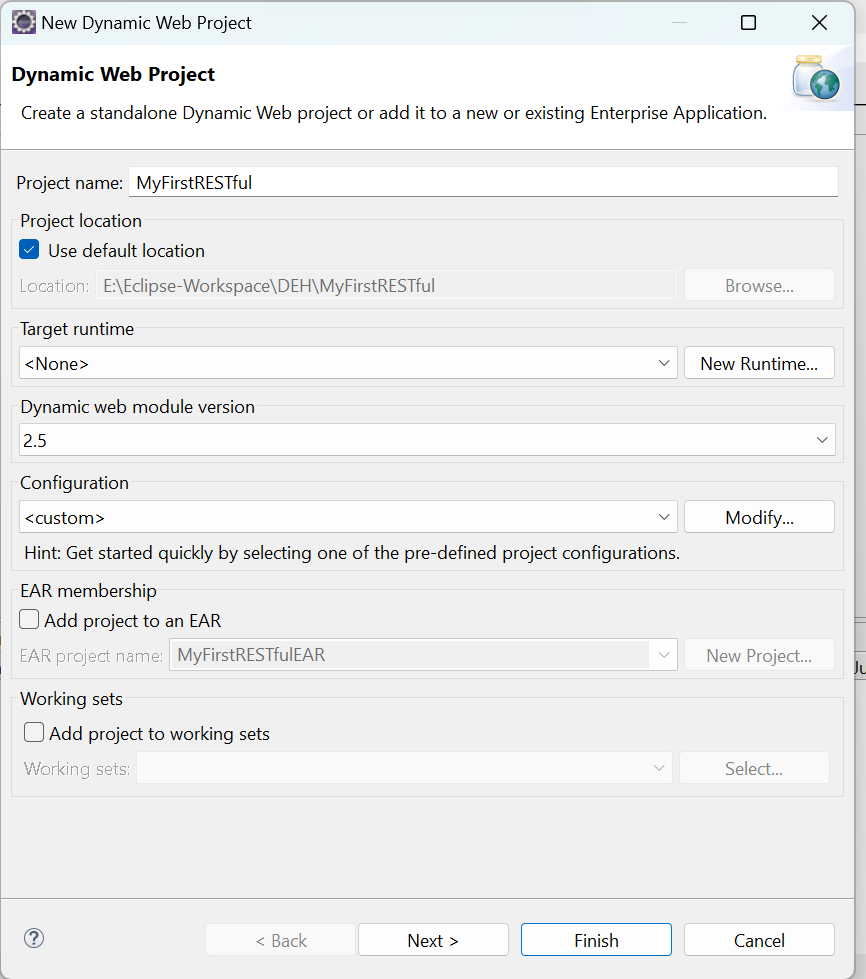
3)
3) Tick mark on “Generate web.xml deployment descriptor” à Finish
4) 4) Expand your project and right click on webcontent folder à New à select JSP File option à Type the file name as index.jsp
5) 5) Open index.jsp and type below code.
index.jsp
<%@ page language="java"
contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD
HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>My first RESTful service</title>
</head>
<body>
<h1>Welcome to my RESTful services</h1>
</body>
</html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
</head>
<body>
<h1>Welcome to my RESTful services</h1>
</body>
</html>
7) 7) Download the jersey-bundle-1.19.1.jar from internet and copy the jar to clipboard. Select your web service project, expand webcontent folder à WEB-INF à right click on lib folder and paste your jar file
Name : jersey-bundle-1.19.1.jar
1) 7) Select your web service project, expand Java Resource and right click on src folder à New à class
RESTfulService.java
package com.isl.deh;
import javax.ws.rs.Consumes;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Response;
@Path("/DEH")
public class RESTfulService {
private String sRespMsg;
private String sResponse;
/***********************************************************
* [Service-1] =
************************************************************/
@POST
@Consumes("text/xml")
@Produces("text/xml")
@Path("/Add")
public Response addNo(
// @FormParam("FirstNo") String sFirstNo,
// @FormParam("SecondNo") String sSecondNo
@QueryParam("First") String sFirstNo,
@QueryParam("Second") String sSecondNo) {
System.out.println("****inside add service****");
try {
System.out.println("****Request Param1=" + sFirstNo);
System.out.println("****Request Param2=" + sSecondNo);
RESTfulDAO dao = new RESTfulDAO();
int a = Integer.parseInt(sFirstNo);
int b = Integer.parseInt(sSecondNo);
int c = dao.addition(a, b);
sResponse = Integer.toString(c);
System.out.println("***Response -->" + sResponse);
} catch (Exception e) {
sRespMsg = e.getMessage();
System.out.println("***Exception-->" + e.getMessage());
return Response.status(404).entity(sRespMsg).build();
}
return Response.status(200).entity(sResponse).build();
}// addNo()
}
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Response;
public class RESTfulService {
private String sResponse;
* [Service-1] =
************************************************************/
@POST
@Consumes("text/xml")
@Produces("text/xml")
@Path("/Add")
public Response addNo(
// @FormParam("FirstNo") String sFirstNo,
// @FormParam("SecondNo") String sSecondNo
@QueryParam("First") String sFirstNo,
@QueryParam("Second") String sSecondNo) {
System.out.println("****Request Param2=" + sSecondNo);
int b = Integer.parseInt(sSecondNo);
System.out.println("***Exception-->" + e.getMessage());
return Response.status(404).entity(sRespMsg).build();
}
return Response.status(200).entity(sResponse).build();
}
package com.isl.deh;
public class RESTfulDAO {
public int addition(int a, int b){
int res = a+b;
return res;
}
}
public int addition(int a, int b){
int res = a+b;
return res;
}
7) Select your web service project, expand webcontent and WEB-INF folder à Open web.xml file and write below code for configuring your java package as RESTful services.
web.xml
<?xml version="1.0"
encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
id="WebApp_ID" version="2.5">
<display-name>RestfulTest</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>REST_Service</servlet-name>
<servlet-class>
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
id="WebApp_ID" version="2.5">
<display-name>RestfulTest</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<servlet-name>REST_Service</servlet-name>
<servlet-class>
com.sun.jersey.spi.container.servlet.ServletContainer
</servlet-class>
<init-param>
<param-name>com.sun.jersey.config.property.packages</param-name>
<param-value>com.isl.deh</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>REST_Service</servlet-name>
<url-pattern>/Service/*</url-pattern>
</servlet-mapping>
</web-app>
<init-param>
<param-name>com.sun.jersey.config.property.packages</param-name>
<param-value>com.isl.deh</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>REST_Service</servlet-name>
<url-pattern>/Service/*</url-pattern>
</servlet-mapping>
</web-app>
Comments
Post a Comment