Array in Java
Array in Java
Java array is an object which contains elements of a similar data type. In Java, array is an object of a dynamically generated class. Java array inherits the Object class, and implements the Serializable as well as Cloneable interfaces. We can store primitive values or objects in an array in Java.
Java provides the feature of anonymous arrays which is not available in C/C++.
Advantages
- Code Optimization: It makes the code optimized, we can retrieve or sort the data efficiently.
- Random access: We can get any data located at an index position.
Disadvantages
- Size Limit: We can store only the fixed size of elements in the array. It doesn't grow its size at runtime. To solve this problem, collection framework is used in Java which grows automatically.
Declaring Array Variables
To use an array in a
program, you must declare a variable to reference the array, and you must
specify the type of array the variable can reference. Here is the syntax for
declaring an array variable −
Syntax
OR
OR
Note − The
style DataType [] arrayRefVar is preferred. The style DataType arrayReferenceVar[] comes
from the C/C++ language and was adopted in Java.
Creating Arrays
You can create an
array by using the new operator with the following syntax −
Syntax
arrayReferenceVar = new DataType[arraySize];
The above statement
does two things −
·
It creates an array using new DataType
[arraySize].
·
It assigns the reference of the newly created array to the variable
arrayReferenceVar.
Declaring an array
variable, creating an array, and assigning the reference of the array to the
variable can be combined in one statement, as shown below −
DataType[] arrayRefenceVar = new DataType[arraySize];
The code snippets of this above 2 syntax −
int []arr=new int[2];
arr[0]=10;
arr[1]=15;
System.out.println(arr[0]);
System.out.println(arr[1]);
Alternative way to create arrays as follows −
DataType[] arrayRefenceVar = {value0, value1, ..., valuek};
The array elements are accessed through the index. Array indices are 0-based; that is, they start from 0 to arrayRefVar.length-1.
Example
for(int i=0;i<name.length;i++){
System.out.println("====="+name[2]);
Example
Assign 1 to 10 numbers in an array and print all elements using for loop
public class ArrayExample2 {
public static void main(String[] args) {
//with the help of Array
int []arr=new int[10];
//assign value to array elements
for(int i=0;i<10;i++){
int number=i+1;
arr[i] = number;
}
System.out.println("Size of array :"+arr.length);
//printing elements of array
for(int i=0;i<10;i++){
System.out.println(arr[i]);
}
}
}
Output
1
2
3
4
5
6
7
8
9
10
The foreach Loops
JDK 1.5 introduced a
new for loop known as foreach loop or enhanced for loop, which enables you to
traverse the complete array sequentially without using an index variable.
Example
The following code
displays all the elements in the array myName −
public
class TestArray2 {
String [] myName =
{"Rahul",
"Papu", "Deepak", "Prabhat"};
for (String name: myName)
{
System.out.println(name);
}
}
}
This will produce the
following result −
Output
Types of Array in java
There are two types of array.
- Single Dimensional Array
- Multidimensional Array
Single Dimensional Array in Java
Syntax to Declare an Array in Java
Multidimensional Array in Java
In such case, data is stored in row and column based index (also known as matrix form).
Syntax to Declare Multidimensional Array in Java
Example to instantiate Multidimensional Array in Java
Output
Processing Arrays
When processing array elements, we often use either for loop or foreach loop because all of the elements in an array are of the same type and the size of the array is known.
Example
Here is a complete example showing how to create, initialize, and process arrays −
Following statement declares an array variable, myList, creates an array of 10 elements of double type and assigns its reference to myList −
double[] myList = new double[10];
Following picture represents array myList. Here, myList holds ten double values and the indices are from 0 to 9.
double[] myList = {1.9, 2.9, 3.4, 3.5};
// Print all the array elements
for (int i = 0; i < myList.length; i++) {
System.out.println(myList[i] + " ");
}
// Summing all elements
double total = 0;
for (int i = 0; i < myList.length; i++) {
total += myList[i];
}
System.out.println("Total is " + total);
// Finding the largest element
double max = myList[0];
for (int i = 1; i < myList.length; i++) {
if (myList[i] > max) max = myList[i];
}
System.out.println("Max is " + max);
}
This will produce the following result −
Output
2.9
3.4
3.5
Total is 11.7
Max is 3.5
Copying a Java Array
We can copy an array to another by the arraycopy() method of System class.
Syntax of arraycopy method
Example of Copying an Array in Java
Output:
caffein
Cloning an Array in Java
Since, Java array implements the Cloneable interface, we can create the clone of the Java array. If we create the clone of a single-dimensional array, it creates the deep copy of the Java array. It means, it will copy the actual value. But, if we create the clone of a multidimensional array, it creates the shallow copy of the Java array which means it copies the references.
Output:
Printing original array: 33 3 4 5 Printing clone of the array: 33 3 4 5 Are both equal? false
Addition of 2 Matrices in Java
Let's see a simple example that adds two matrices.
Output:
2 6 8 6 8 10
Multiplication of 2 Matrices in Java
In the case of matrix multiplication, a one-row element of the first matrix is multiplied by all the columns of the second matrix which can be understood by the image given below.
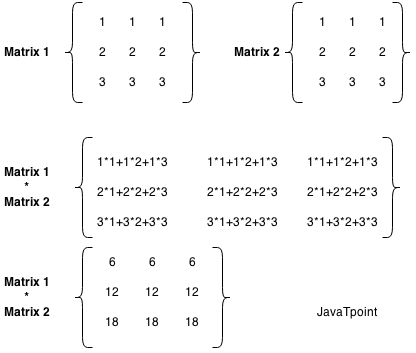
Let's see a simple example to multiply two matrices of 3 rows and 3 columns.
Output:
6 6 6 12 12 12 18 18 18
ArrayIndexOutOfBoundsException
The Java Virtual Machine (JVM) throws an ArrayIndexOutOfBoundsException if length of the array in negative, equal to the array size or greater than the array size while traversing the array.
//Java Program to demonstrate the case of
//ArrayIndexOutOfBoundsException in a Java Array.
public class TestArrayException{
public static void main(String args[]){
int arr[]={10,20,20,40};
for(int i=0;i<=arr.length;i++){
System.out.println(arr[i]);
}
}
}
Output:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 4 at TestArrayException.main(TestArrayException.java:5) 10 20 30 40
Comments
Post a Comment