Difference between String and StringBuffer
String
Strings are constant; their values cannot be changed after they are created. String buffers support mutable strings. Because String objects are immutable they can be shared. For example:
String str = new String(data);
Here are some more examples of how strings can be used:
System.out.println("abc");
String cde = "cde";
System.out.println("abc" + cde);
String c = "abc".substring(2,3);
String d = cde.substring(1, 2);
Example of String
Output:
Chennai ChennaiMumbai Bhubaneswar
StringBuffer
StringBuffer is
class available in java, A thread-safe, mutable sequence of characters. A
string buffer is like a String, but can be modified. At any point in time it
contains some particular sequence of characters, but the length and content of
the sequence can be changed through certain method calls.
String
buffers are safe for use by multiple threads. The methods are synchronized
where necessary so that all the operations on any particular instance behave as
if they occur in some serial order that is consistent with the order of the
method calls made by each of the individual threads involved.
The
principal operations on a StringBuffer are the append and insert methods,
which are overloaded so as to accept data of any type. Each effectively
converts a given datum to a string and then appends or inserts the characters
of that string to the string buffer. The append method always adds
these characters at the end of the buffer; the insert method adds the
characters at a specified point.
For
example, if z refers to a string buffer object whose current contents
are "start", then the method call z.append("le") would
cause the string buffer to contain "startle", whereas z.insert(4,
"le") would alter the string buffer to contain "starlet".
In
general, if sb refers to an instance of a StringBuffer, then sb.append(x) has
the same effect as sb.insert(sb.length(), x).
Whenever
an operation occurs involving a source sequence (such as appending or inserting
from a source sequence) this class synchronizes only on the string buffer performing
the operation, not on the source.
Every
string buffer has a capacity. As long as the length of the character sequence
contained in the string buffer does not exceed the capacity, it is not
necessary to allocate a new internal buffer array. If the internal buffer
overflows, it is automatically made larger. As of release JDK 5, this class has
been supplemented with an equivalent class designed for use by a single
thread, StringBuilder. The StringBuilder class
should generally be used in preference to this one, as it supports all of the
same operations but it is faster, as it performs no synchronization.
Example of StringBuffer
Output:
Mumbai
Mumbai City
Mumbai Great City
Difference between String and StringBuffer
There are many differences between String and StringBuffer. A list of differences between String and StringBuffer are given below:
No. | String | StringBuffer |
---|---|---|
1) | The String class is immutable. | The StringBuffer class is mutable. |
2) | String is slow and consumes more memory when we concatenate too many strings because every time it creates new instance. | StringBuffer is fast and consumes less memory when we concatenate t strings. |
3) | String class overrides the equals() method of Object class. So you can compare the contents of two strings by equals() method. | StringBuffer class doesn't override the equals() method of Object class. |
4) | String class is slower while performing concatenation operation. | StringBuffer class is faster while performing concatenation operation. |
5) | String class uses String constant pool. | StringBuffer uses Heap memory |
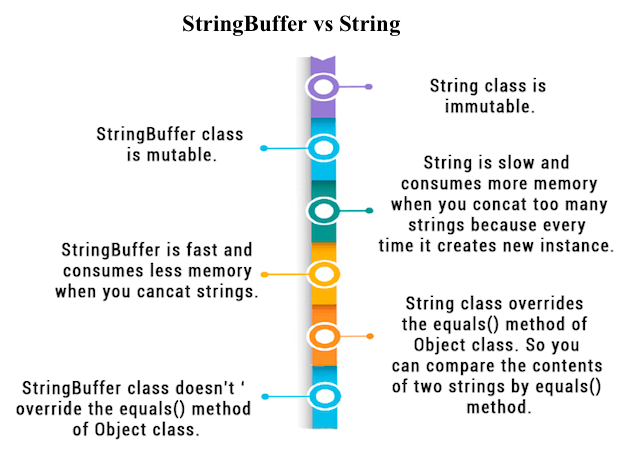
Performance Test of String and StringBuffer
ConcatTest1.java
Output:
Time taken for concating a value in StringBuffer: 0ms
The above code, calculates the time required for concatenating a string using the String class and StringBuffer class.
String and StringBuffer HashCode Test
As we can see in the program given below, String returns new hashcode while performing concatenation but the StringBuffer class returns same hashcode.
InstanceTest.java
Output:
Hashcode test of String: 3254818 229541438 Hashcode test of StringBuffer: 118352462 118352462
Comments
Post a Comment